Android
Location Permission Access
When instantiating the Android booking screen make sure the user has granted location permissions. If these haven't been granted then the user will be unable to use the map to select an address and the pickup address will not default to the user's location.
When instantiating the Android booking screen make sure the user has granted location permissions. If these are granted, then the pickup address will default to the users location and the user will be able to select a pick up or drop off point using the map as well as searching for an address. If these permissions haven't been granted then the user will be unable to use the map to select an address and the pickup address will not default to the user's location.
To initiate the callback flow for the Android UI SDK, construct the intent with the method buildForOnActivityResultCallback(context: Context): Intent
instead of the usual build(context: Context)
method and pass this into startActivityForResult()
with your own request code. When the activity returns to your onActivityResult(requestCode: Int, resultCode: Int, data: Intent?)
method, you will be able to retrieve the TripInfo
object by calling data?.getParcelableExtra<TripInfo>(BookingCodes.BOOKED_TRIP)
.
Android Booking Screen
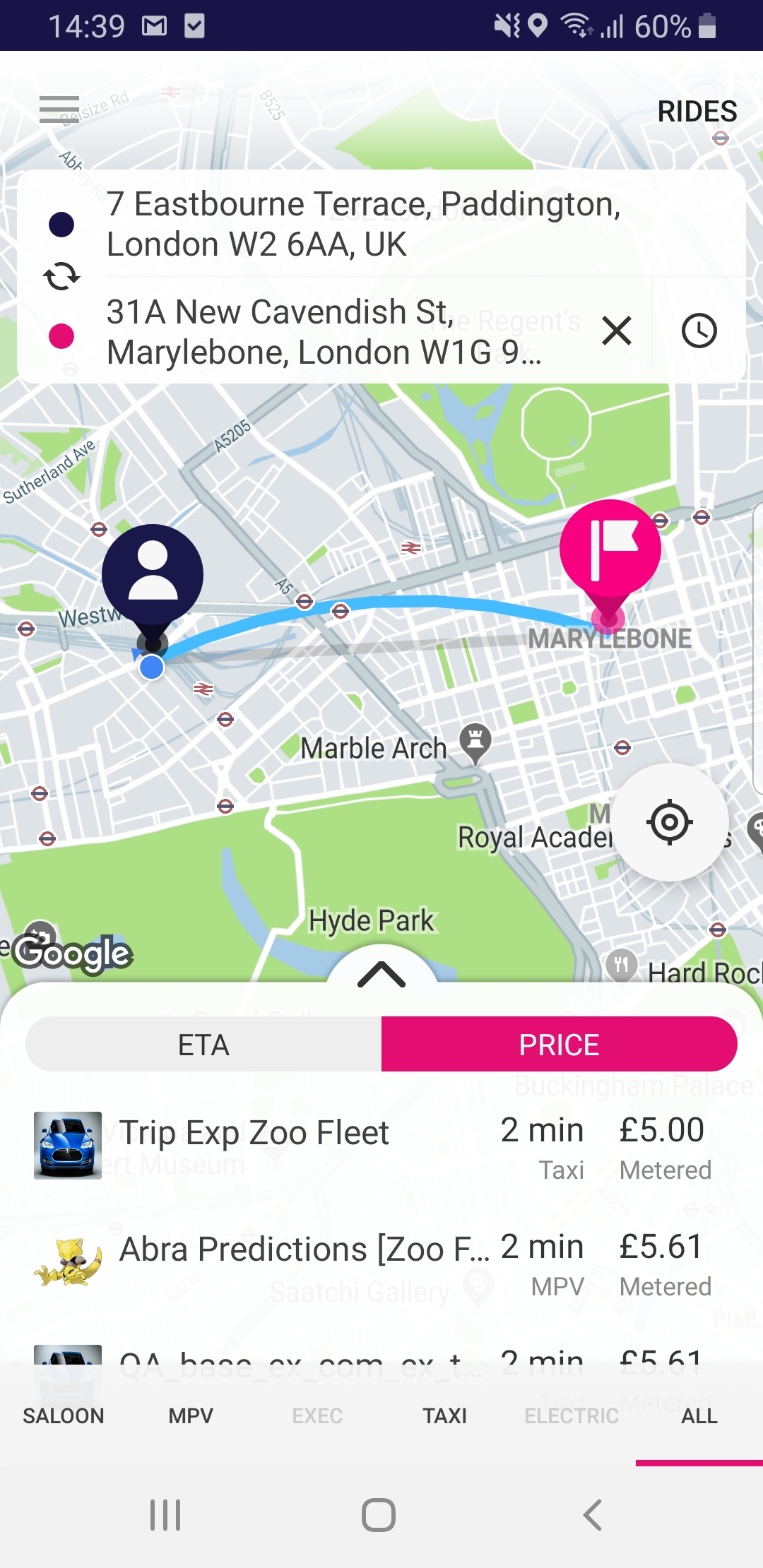
Android Booking Screen
Android example
// launching for primary flow
val intent = BookingActivity.Builder.builder
.initialLocation(location)
.build(this)
startActivity(intent)
// launching for callback example
val MY_REQ_CODE = 101
val callbackIntent = BookingActivity.Builder.builder
.initialLocation(location)
.buildForOnActivityResultCallback(this)
startActivityForResult(callbackIntent, MY_REQ_CODE)
// receiving booked trip for callback flow
override fun onActivityResult(requestCode: Int, resultCode: Int, data: Intent?) {
if (resultCode == Activity.RESULT_OK && requestCode == MY_REQ_CODE) {
val bookedTrip = data?.getParcelableExtra<TripInfo>(BookingCodes.BOOKED_TRIP)
// do something with bookedTrip
}
super.onActivityResult(requestCode, resultCode, data)
}
Builder variables
Variable | Description |
---|---|
tripDetails : TripInfo | The activity will take the origin and destination, if available from tripDetails , use this to pre-populate the addressview and begin fetching quotes |
outboundTripId : String | The outboundTripId is expected when the trip is booked from a ‘rebook’ button in another activity, it’s used for analytics purposes only |
initialLocation : Location | If an initialLocation is passed in the activity will zoom straight to this without having to wait for the device to return a location |
Booking Details
Android Booking Details Screen
When an authenticated user selects a quote, they are then taken to the booking details screen which enables them to add or update their payment details and make the booking.
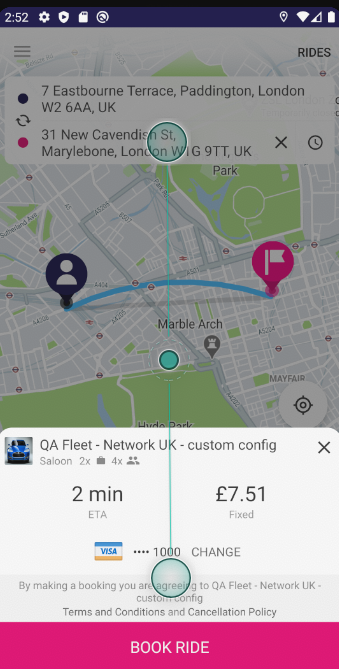
Android Booking Details Screen
Android Guest Booking Details Screen
When a guest user selects a quote, they are then taken to the guest booking details screen which enables them to add their booking details i.e. first name, last name, email address, phone number and payment details. The Book Ride button is disabled until all mandatory fields have been completed. There are also optional comments and flight number (where applicable) fields.
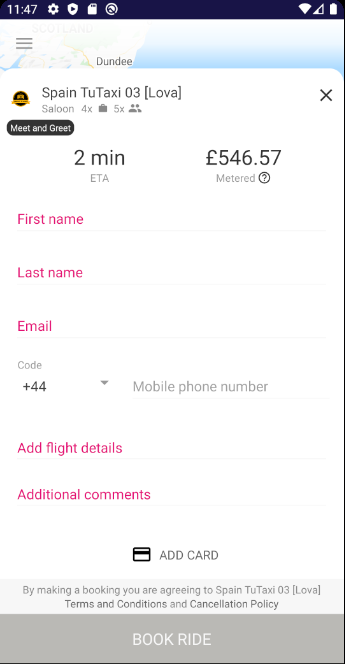
Android Guest Booking Details Screen